Another cute little new feature of iOS 6 is the built-in pull-to-refresh control - UIRefreshControl
. It is really simple to wire up with Xamarin on a regular UITableViewController or with MonoTouch.Dialog.
In case you're unfamiliar with it, this is how the new control looks:
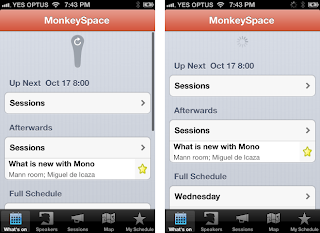
To implement:
- Assign the RefreshControl property of a UITableViewController to a new instance of the control, eg.
RefreshControl = new UIRefreshControl();
- Create a handler for the control's ValueChanged event, eg
RefreshControl.ValueChanged += HandleValueChanged;
. In this handler your code will do whatever is required to refresh the data when the user pulls down enough to trigger the event. - In
HandleValueChanged
code, once you have refreshed the table, call the EndRefreshing method of the control to stop it spinning. Your refresh code was probably not running on the main thread, in which case you'll probably want to use InvokeOnMainThread like this:InvokeOnMainThread (() => {RefreshControl.EndRefreshing (); });
There are additional features you may use:
BeginRefreshing
- call this method from your code if you start a refresh operation from code (eg. on a timer or in response to some other event). This sets the UI of the control to indicate there is already a refresh in progress.Refreshing
- whether there is already a refresh in progress.AttributedTitle
- optional text that appears under the refresh control.TintColor
- customize the appearance of the control to match your application's theme.
Refer to Apple's UIRefreshControl doc for additional info.
UPDATE: What about iOS 5?This above example will not work on earlier versions of iOS - the UIRefreshControl
class does not exist, nor does the RefreshControl
property on UITableViewController
. To get around this problem, the following code does a system check and falls back to an old-fashioned navbarbutton in earlier versions of iOS:
if (UIDevice.CurrentDevice.CheckSystemVersion (6,0)) { // UIRefreshControl iOS6 RefreshControl = new UIRefreshControl(); RefreshControl.ValueChanged += (sender, e) => { Refresh(); }; } else { // old style refresh button NavigationItem.SetRightBarButtonItem (new UIBarButtonItem (UIBarButtonSystemItem.Refresh), false); NavigationItem.RightBarButtonItem.Clicked += (sender, e) => { Refresh(); }; }
The Refresh
method should contain the code that actually gets new data and updates the UITableView
. That method should contain a similar if (CheckSystemVersion(6,0))
clause that wraps the call to the RefreshControl.EndRefreshing
method on the main thread. Users on older operating systems will see this:
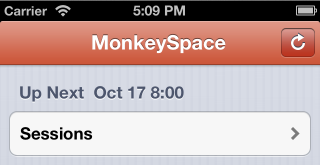